AEM Screens is built on the solid foundation of AEM Sites and enables marketers and IT personnel to create and manage experiences on multiple digital screens.
AEM Screens is a powerful web-based solution that allows you to create dedicated digital menu boards to expand customer interaction and deliver unified and useful brand experiences into physical venues.
AEM Screen are useful in stores, hotels, banks, healthcare and educational institutions, and many more - from the same AEM platform.
Content for AEM Screens is managed in channels .
AEM Screens Player renders content present within channels onto displays.
The following figure defines the personas and their roles for AEM Screens:
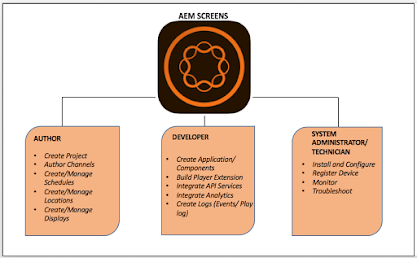
The following steps allow you to create a sample project for Screens and publish content to Screens player Application :
Step 1: AEM Screen player Installation
Download AEM Screen player from below URL:
https://download.macromedia.com/screens/
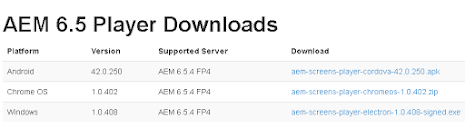
Note: It Supports Android, Chrome OS and Windows to work with latest version of AEM.
You will see a following console Upon starting of the Application:
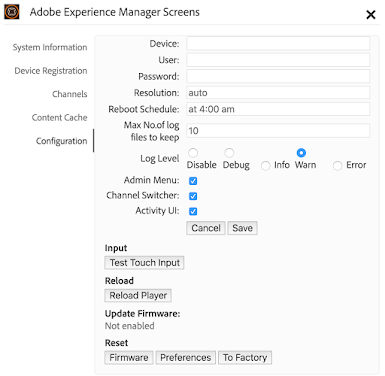
Step 2: Make the required AEM configuration changes
Open Adobe Experience Manager Web Console Configuration using below URL:
https://localhost:4502/system/console/configMgr
Configuration 1: Search for Apache sling referrer filter configuration and check the Allow Empty value to true.
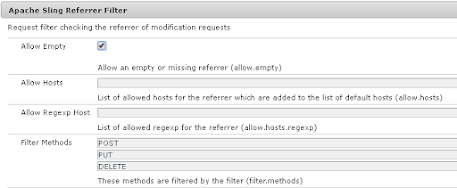
Configuration 2: Search for Apache Felix Jetty Based HTTP Service Check the ENABLE HTTP option, as shown in the figure below:
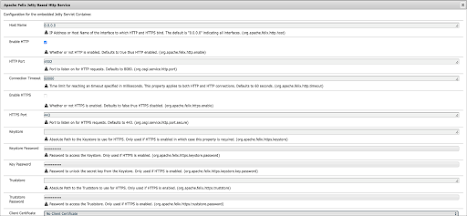
Step 3: Creating New AEM Screens Project
Go to, http://localhost:4502/screens.html/content/screens or select Screens from AEM start navigation.
Select Create à Create project
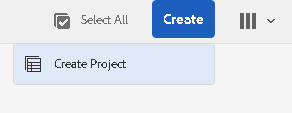
Select Screens and select next.
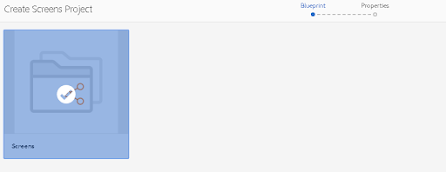
Enter the project Title and select Create.
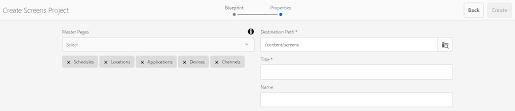
Following project structure will be created:
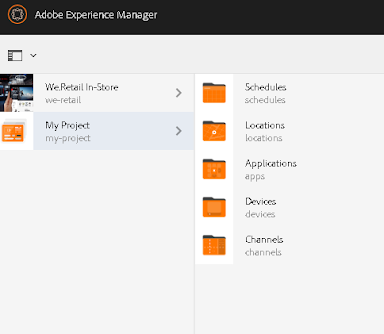
Step 4: Device Registration
Select Device from project structure and from options select device manager or go to, http://localhost:4502/screens/devices.html/content/screens/my-project/devices
Select Device registration:

In the next step you will see a list of pending devices on which you have installed and AEM Screen application which are on same server:

Select this device and click on Register device.
It will search for the device and its configuration
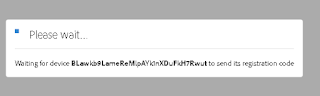
Go to your Desktop/Android application if the registration code matched, your device will be successfully registered/Assigned with this project.

Step 5: Next step is to assign display to assigned device from the different available locations:

for example - /content/screens/we-retail/locations/demo/flagship/single
This Display will be mapped to Your AEM Screen App and will be activated on it.